♣ Whenever we want to execute a single statement or block of statements based on some condition then we will implement conditional statements
♣ In C#.net we can implement two types of conditional statements
1. IF Condition
2. Switch Condition
♣ If Condition we can implement in 3 ways
1. IF
2. IF Else
3. IF Else IF
# IF :
♣ Syntax is like below
if(condition)
{
statement 1;
statement 2;
}
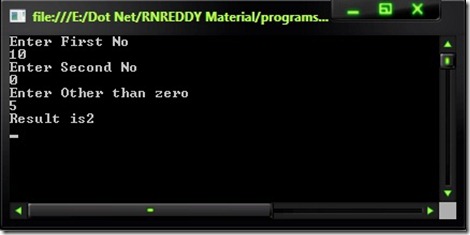
# IF Else :
♣ Syntax is like below
if(condition)
{
Statement 1;
}
else
{
Statement 2;
}
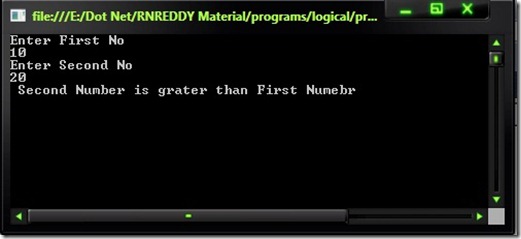
# IF Else IF :
♣ Syntax is Like Below
if(condition)
statement 1;
else if(condition 2)
statement 2;
else if(condition 3)
statement 3;
else
statement 4;
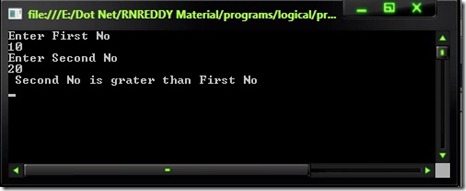
♣ Whenever we want to execute single case among multiple cases we will go for switch condition
♣ Syntax is like below
Switch(<expression>)
{
case 1:
statement 1;
break;
case 2:
statement 2;
break;
case 3:
statement 3;
break;
Default :
statement 4;
break;
}
Note : Break is a C#.Net keyword, once break statement is executed control will not execute any other statements with in the switch block, it will transfer to out of the switch block.
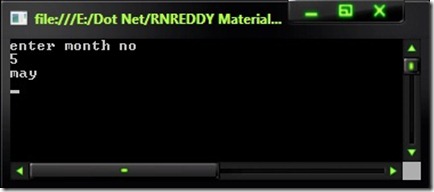
♣ In C#.net we can implement two types of conditional statements
1. IF Condition
2. Switch Condition
IF Condition :
1. IF
2. IF Else
3. IF Else IF
# IF :
♣ Syntax is like below
if(condition)
{
statement 1;
statement 2;
}
Example for Just IF
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro9
{
class Program
{
static void Main(string[] args)
{
/*simple if */
Console.WriteLine("Enter First No");
int n1 = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter Second No");
int n2 = Convert.ToInt32(Console.ReadLine());
if (n2==0)
{
Console.WriteLine("Enter Other than zero");
n2 = Convert.ToInt32(Console.ReadLine());
}
int res = n1 / n2;
Console.WriteLine("Result is"+res);
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro9
{
class Program
{
static void Main(string[] args)
{
/*simple if */
Console.WriteLine("Enter First No");
int n1 = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter Second No");
int n2 = Convert.ToInt32(Console.ReadLine());
if (n2==0)
{
Console.WriteLine("Enter Other than zero");
n2 = Convert.ToInt32(Console.ReadLine());
}
int res = n1 / n2;
Console.WriteLine("Result is"+res);
Console.ReadLine();
}
}
}
Output :
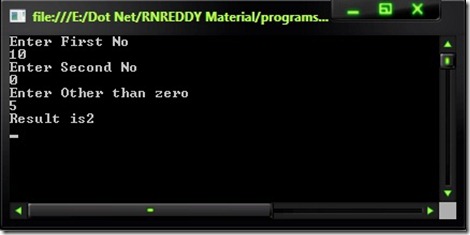
# IF Else :
♣ Syntax is like below
if(condition)
{
Statement 1;
}
else
{
Statement 2;
}
Example to compare two numbers
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro10
{
class Program
{
static void Main(string[] args)
{
/*simple if else*/
Console.WriteLine("Enter First No");
int i = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter Second No");
int j = Convert.ToInt32(Console.ReadLine());
if (i > j)
Console.WriteLine("First Numebr is grater than Second Number");
else
Console.WriteLine(" Second Number is grater than First Numebr");
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro10
{
class Program
{
static void Main(string[] args)
{
/*simple if else*/
Console.WriteLine("Enter First No");
int i = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter Second No");
int j = Convert.ToInt32(Console.ReadLine());
if (i > j)
Console.WriteLine("First Numebr is grater than Second Number");
else
Console.WriteLine(" Second Number is grater than First Numebr");
Console.ReadLine();
}
}
}
Output :
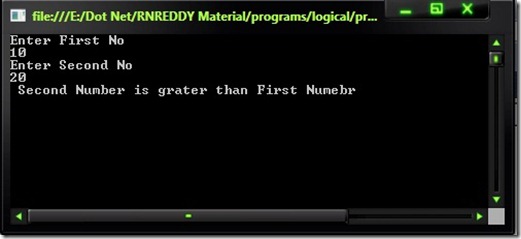
# IF Else IF :
♣ Syntax is Like Below
if(condition)
statement 1;
else if(condition 2)
statement 2;
else if(condition 3)
statement 3;
else
statement 4;
Example for IF Else If
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro11
{
class Program
{
static void Main(string[] args)
{
/*if else if which is grater no */
Console.WriteLine("Enter First No");
int i = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter Second No");
int j = Convert.ToInt32(Console.ReadLine());
if (i > j)
Console.WriteLine("First No is grater than Second No");
else if (i < j)
Console.WriteLine(" Second No is grater than First No");
else
Console.WriteLine("First No, Second No both are equal");
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro11
{
class Program
{
static void Main(string[] args)
{
/*if else if which is grater no */
Console.WriteLine("Enter First No");
int i = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter Second No");
int j = Convert.ToInt32(Console.ReadLine());
if (i > j)
Console.WriteLine("First No is grater than Second No");
else if (i < j)
Console.WriteLine(" Second No is grater than First No");
else
Console.WriteLine("First No, Second No both are equal");
Console.ReadLine();
}
}
}
Output :
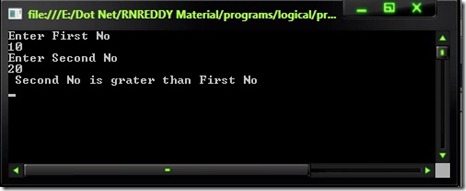
Switch Condition :
♣ Syntax is like below
Switch(<expression>)
{
case 1:
statement 1;
break;
case 2:
statement 2;
break;
case 3:
statement 3;
break;
Default :
statement 4;
break;
}
Note : Break is a C#.Net keyword, once break statement is executed control will not execute any other statements with in the switch block, it will transfer to out of the switch block.
Example for Switch Condition
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro12
{
class Program
{
static void Main(string[] args)
{
/*example of switch case month no to name*/
Console.WriteLine("enter month no");
int no = int.Parse(Console.ReadLine());
switch (no)
{
case 1: Console.WriteLine("jan");
break;
case 2: Console.WriteLine("feb");
break;
case 3: Console.WriteLine("mar");
break;
case 4: Console.WriteLine("apr");
break;
case 5: Console.WriteLine("may");
break;
case 6: Console.WriteLine("jun");
break;
case 7: Console.WriteLine("jul");
break;
case 8: Console.WriteLine("aug");
break;
case 9: Console.WriteLine("sec");
break;
case 10: Console.WriteLine("oct");
break;
case 11: Console.WriteLine("nov");
break;
case 12: Console.WriteLine("dec");
break;
default: Console.WriteLine(" enter no 1-12 only");
break;
}
Console.ReadLine();
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace pro12
{
class Program
{
static void Main(string[] args)
{
/*example of switch case month no to name*/
Console.WriteLine("enter month no");
int no = int.Parse(Console.ReadLine());
switch (no)
{
case 1: Console.WriteLine("jan");
break;
case 2: Console.WriteLine("feb");
break;
case 3: Console.WriteLine("mar");
break;
case 4: Console.WriteLine("apr");
break;
case 5: Console.WriteLine("may");
break;
case 6: Console.WriteLine("jun");
break;
case 7: Console.WriteLine("jul");
break;
case 8: Console.WriteLine("aug");
break;
case 9: Console.WriteLine("sec");
break;
case 10: Console.WriteLine("oct");
break;
case 11: Console.WriteLine("nov");
break;
case 12: Console.WriteLine("dec");
break;
default: Console.WriteLine(" enter no 1-12 only");
break;
}
Console.ReadLine();
}
}
}
Output :
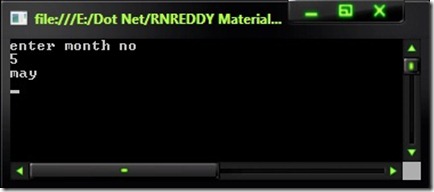